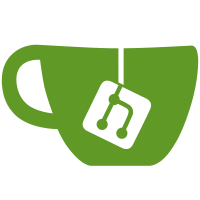
6 changed files with 232 additions and 12 deletions
@ -0,0 +1,39 @@ |
|||||||
|
{% extends "ordr2:templates/layout.jinja2" %} |
||||||
|
|
||||||
|
{% block title %} Ordr | Login Failed {% endblock title %} |
||||||
|
|
||||||
|
{% block content %} |
||||||
|
<div class="row justify-content-center"> |
||||||
|
<div class="col-8"> |
||||||
|
|
||||||
|
<h1>Try again…</h1> |
||||||
|
<p class="alert alert-danger">You entered the wrong username or password.</p> |
||||||
|
|
||||||
|
<form action="{{ request.resource_url(request.root, 'account', 'login') }}" method="POST" id="login-form"> |
||||||
|
<input type="hidden" name="csrf_token" value="{{ get_csrf_token() }}"> |
||||||
|
<div class="form-group row"> |
||||||
|
<label for="username" class="col-2">Username</label> |
||||||
|
<div class="col-6"> |
||||||
|
<input name="username" id="username" type="text" class="form-control"> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
<div class="form-group row"> |
||||||
|
<label for="password" class="col-2">Password</label> |
||||||
|
<div class="col-6"> |
||||||
|
<input name="password" id="password>" type="password" class="form-control"> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
<div class="form-group row"> |
||||||
|
<div class="col-2"></div> |
||||||
|
<div class="col-6"> |
||||||
|
<button type="submit" class="btn btn-sm btn-primary">Log in</button> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
</form> |
||||||
|
|
||||||
|
<p> |
||||||
|
If you forgot your password you can <a href="{{ request.resource_url(context, 'forgot-password') }}">reset it</a>. |
||||||
|
</p> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
{% endblock content %} |
Reference in new issue