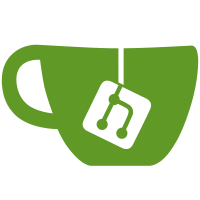
5 changed files with 69 additions and 4 deletions
@ -1 +1 @@
@@ -1 +1 @@
|
||||
$(document).ready(function() {
function capitalize(s){
return s.replace( /\b./g, function(a){ return a.toUpperCase(); } );
};
function generate_user_name() {
var first_name = $('.registration .item-first_name input').val();
var last_name = $('.registration .item-last_name input').val();
var user_name = capitalize(first_name) + capitalize(last_name);
return user_name.replace( /\s/g, '')
};
// autocomplete of the username (registration form)
$('.registration .item-user_name input').attr('disabled', 'disabled');
$('.registration .item-user_name input').val( generate_user_name() );
$('.registration .item-first_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration .item-last_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration #deformCreate_Account').click(function() {
$('.registration .item-user_name input').removeAttr('disabled');
return true;
});
// "dispatch" clicking a th to the corresponding anchor
$('th.sortable').click( function() {
window.location = $(this).children('a').attr('href');
});
// "dispatch" clicking list item in collapse to the corresponding anchor
$('.accordion li').click( function() {
window.location = $(this).children('a').attr('href');
});
// the mark all
$('input[name="mark_all"]').click(function() {
var checked_status = this.checked;
$('input[name*="marked"]').each(function() {
this.checked = checked_status;
});
if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':hidden') ) {
$('.marking-needed').fadeIn("fast");
} else if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':visible') ) {
// do nothing
} else {
$('.marking-needed').fadeOut("fast");
}
});
// show actions only if some data is marked
$('input[name*="marked"]').change(function() {
if( $('input[name*="marked"]').is(':checked') ) {
if( $('.marking-needed').is(':hidden') )
$('.marking-needed').fadeIn("slow");
} else {
$('.marking-needed').fadeOut("slow");
}
});
// quick-actions
$('.quick-action a[data-value]').click(function(event) {
event.preventDefault();
var value = $(this).attr('data-value');
var action = $(this).closest('div').attr('data-action');
$('select[name*='+action+']').each(function() {
$(this).val(value);
});
});
// submit search
$('.search-form input[type="search"]').keypress(function(event) {
if( event.keyCode == 13 && $('.typeahead.dropdown-menu').is(':hidden') )
$('.search-form').submit();
});
// aside filter
$('.filter input[type="checkbox"]').click( function() {
if( $(this).is(':checked') ) {
$(this).parents('li').addClass('active');
} else {
$(this).parents('li').removeClass('active');
}
});
// tooltips
$('.page-controls').tooltip({
selector: "[rel=tooltip]"
});
// calculator
if ( $('input[name="price_unit"]').length ) {
if( $('input[name="price_unit"]').val() != '' && $('input[name="quantity"]').val() != '' ){
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
}
$('input[name="price_unit"], input[name="quantity"]').keyup(function() {
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
});
}
if ( $('input[name="currency"]').length ) {
// added currency to total price
if( $('input[name="currency"]').val() != '' ){
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
}
$('input[name="currency"]').keyup(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
$('input[name="currency"]').change(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
}
}); |
||||
$(document).ready(function() {
function capitalize(s){
return s.replace( /\b./g, function(a){ return a.toUpperCase(); } );
};
function generate_user_name() {
var first_name = $('.registration .item-first_name input').val();
var last_name = $('.registration .item-last_name input').val();
var user_name = capitalize(first_name) + capitalize(last_name);
return user_name.replace( /\s/g, '')
};
// autocomplete of the username (registration form)
$('.registration .item-user_name input').val( generate_user_name() );
$('.registration .item-first_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration .item-last_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration #deformCreate_Account').click(function() {
$('.registration .item-user_name input').removeAttr('disabled');
return true;
});
// "dispatch" clicking a th to the corresponding anchor
$('th.sortable').click( function() {
window.location = $(this).children('a').attr('href');
});
// "dispatch" clicking list item in collapse to the corresponding anchor
$('.accordion li').click( function() {
window.location = $(this).children('a').attr('href');
});
// the mark all
$('input[name="mark_all"]').click(function() {
var checked_status = this.checked;
$('input[name*="marked"]').each(function() {
this.checked = checked_status;
});
if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':hidden') ) {
$('.marking-needed').fadeIn("fast");
} else if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':visible') ) {
// do nothing
} else {
$('.marking-needed').fadeOut("fast");
}
});
// show actions only if some data is marked
$('input[name*="marked"]').change(function() {
if( $('input[name*="marked"]').is(':checked') ) {
if( $('.marking-needed').is(':hidden') )
$('.marking-needed').fadeIn("slow");
} else {
$('.marking-needed').fadeOut("slow");
}
});
// quick-actions
$('.quick-action a[data-value]').click(function(event) {
event.preventDefault();
var value = $(this).attr('data-value');
var action = $(this).closest('div').attr('data-action');
$('select[name*='+action+']').each(function() {
$(this).val(value);
});
});
// submit search
$('.search-form input[type="search"]').keypress(function(event) {
if( event.keyCode == 13 && $('.typeahead.dropdown-menu').is(':hidden') )
$('.search-form').submit();
});
// aside filter
$('.filter input[type="checkbox"]').click( function() {
if( $(this).is(':checked') ) {
$(this).parents('li').addClass('active');
} else {
$(this).parents('li').removeClass('active');
}
});
// tooltips
$('.page-controls').tooltip({
selector: "[rel=tooltip]"
});
// calculator
if ( $('input[name="price_unit"]').length ) {
if( $('input[name="price_unit"]').val() != '' && $('input[name="quantity"]').val() != '' ){
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
}
$('input[name="price_unit"], input[name="quantity"]').keyup(function() {
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
});
}
if ( $('input[name="currency"]').length ) {
// added currency to total price
if( $('input[name="currency"]').val() != '' ){
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
}
$('input[name="currency"]').keyup(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
$('input[name="currency"]').change(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
}
}); |
@ -0,0 +1,42 @@
@@ -0,0 +1,42 @@
|
||||
<div tal:define=" |
||||
name name|field.name; |
||||
oid oid|field.oid; |
||||
style style|field.widget.style; |
||||
size size|field.widget.size; |
||||
css_class css_class|field.widget.css_class; |
||||
unicode unicode|str; |
||||
optgroup_class optgroup_class|field.widget.optgroup_class; |
||||
multiple multiple|field.widget.multiple;" |
||||
tal:omit-tag=""> |
||||
|
||||
<input type="hidden" name="__start__" value="${name}:sequence" |
||||
tal:condition="multiple" /> |
||||
<select tal:attributes=" |
||||
name name; |
||||
id oid; |
||||
class string: form-control ${css_class or ''}; |
||||
multiple multiple; |
||||
size size; |
||||
style style;" |
||||
disabled="disabled"> |
||||
<tal:loop tal:repeat="item values"> |
||||
<optgroup tal:condition="isinstance(item, optgroup_class)" |
||||
tal:attributes="label item.label"> |
||||
<option tal:repeat="(value, description) item.options" |
||||
tal:attributes=" |
||||
selected python:field.widget.get_select_value(cstruct, value); |
||||
class css_class; |
||||
label field.widget.long_label_generator and description; |
||||
value value" |
||||
tal:content="field.widget.long_label_generator and field.widget.long_label_generator(item.label, description) or description"/> |
||||
</optgroup> |
||||
<option tal:condition="not isinstance(item, optgroup_class)" |
||||
tal:attributes=" |
||||
selected python:field.widget.get_select_value(cstruct, item[0]); |
||||
class css_class; |
||||
value item[0]">${item[1]}</option> |
||||
</tal:loop> |
||||
</select> |
||||
<input type="hidden" name="__end__" value="${name}:sequence" |
||||
tal:condition="multiple" /> |
||||
</div> |
@ -0,0 +1,22 @@
@@ -0,0 +1,22 @@
|
||||
<span tal:define="name name|field.name; |
||||
css_class css_class|field.widget.css_class; |
||||
oid oid|field.oid; |
||||
mask mask|field.widget.mask; |
||||
mask_placeholder mask_placeholder|field.widget.mask_placeholder; |
||||
style style|field.widget.style; |
||||
" |
||||
tal:omit-tag=""> |
||||
<input type="text" name="${name}" value="${cstruct}" |
||||
tal:attributes="class string: form-control ${css_class or ''}; |
||||
style style" |
||||
id="${oid}" |
||||
disabled="disabled"/> |
||||
<script tal:condition="mask" type="text/javascript"> |
||||
deform.addCallback( |
||||
'${oid}', |
||||
function (oid) { |
||||
$("#" + oid).mask("${mask}", |
||||
{placeholder:"${mask_placeholder}"}); |
||||
}); |
||||
</script> |
||||
</span> |
Reference in new issue