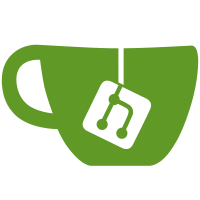
14 changed files with 445 additions and 12 deletions
@ -0,0 +1,70 @@ |
|||||||
|
import colander |
||||||
|
import deform |
||||||
|
|
||||||
|
from ordr2.models import Category |
||||||
|
|
||||||
|
from . import CSRFSchema |
||||||
|
|
||||||
|
CATEGORIES = [(c.name, c.value.capitalize()) for c in Category] |
||||||
|
|
||||||
|
# schema for user registration |
||||||
|
|
||||||
|
class ConsumableSchema(CSRFSchema): |
||||||
|
''' edit or add consumable ''' |
||||||
|
|
||||||
|
cas_description = colander.SchemaNode( |
||||||
|
colander.String() |
||||||
|
) |
||||||
|
category = colander.SchemaNode( |
||||||
|
colander.String(), |
||||||
|
widget=deform.widget.SelectWidget(values=CATEGORIES) |
||||||
|
) |
||||||
|
catalog_nr = colander.SchemaNode( |
||||||
|
colander.String() |
||||||
|
) |
||||||
|
vendor = colander.SchemaNode( |
||||||
|
colander.String() |
||||||
|
) |
||||||
|
package_size = colander.SchemaNode( |
||||||
|
colander.String() |
||||||
|
) |
||||||
|
unit_price = colander.SchemaNode( |
||||||
|
colander.Decimal(), |
||||||
|
widget=deform.widget.MoneyInputWidget() |
||||||
|
) |
||||||
|
currency = colander.SchemaNode( |
||||||
|
colander.String(), |
||||||
|
default='EUR' |
||||||
|
) |
||||||
|
comment = colander.SchemaNode( |
||||||
|
colander.String(), |
||||||
|
widget=deform.widget.TextAreaWidget(rows=5), |
||||||
|
missing='' |
||||||
|
) |
||||||
|
|
||||||
|
@classmethod |
||||||
|
def as_form(cls, request, **override): |
||||||
|
is_new_consumable = override.pop('is_new_consumable', False) |
||||||
|
if is_new_consumable: |
||||||
|
settings = { |
||||||
|
'buttons': ( |
||||||
|
deform.Button(name='save', title='Add Consumable'), |
||||||
|
deform.Button(name='cancel', title='Cancel') |
||||||
|
), |
||||||
|
'css_class': 'form-horizontal', |
||||||
|
} |
||||||
|
else: |
||||||
|
settings = { |
||||||
|
'buttons': ( |
||||||
|
deform.Button(name='save', title='Save changes'), |
||||||
|
deform.Button( |
||||||
|
name='delete', |
||||||
|
title='Delete Consumable', |
||||||
|
css_class='btn-danger' |
||||||
|
), |
||||||
|
deform.Button(name='cancel', title='Cancel') |
||||||
|
), |
||||||
|
'css_class': 'form-horizontal', |
||||||
|
} |
||||||
|
settings.update(override) |
||||||
|
return super().as_form(request, **settings) |
@ -0,0 +1,70 @@ |
|||||||
|
{% extends "ordr2:templates/layout.jinja2" %} |
||||||
|
{% import 'ordr2:templates/macros.jinja2' as macros with context %} |
||||||
|
|
||||||
|
{% block subtitle %} Admin | Consumable | Confirm Delete {% endblock subtitle %} |
||||||
|
|
||||||
|
{% block content %} |
||||||
|
<div class="content controls"> |
||||||
|
|
||||||
|
<div class="container-fluid"> |
||||||
|
<div class="row-fluid"> |
||||||
|
<div class="page-controls"> |
||||||
|
<h1>Delete Consumable{{ 's' if consumables|length > 1 }}</h1> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
|
||||||
|
<div class="row"> |
||||||
|
<div class="span10"> |
||||||
|
|
||||||
|
<div class="action-header"> |
||||||
|
<h3>The following consumable{{ 's' if consumables|length > 1 }} will be deleted:</h3> |
||||||
|
</div> |
||||||
|
|
||||||
|
<form action="{{ request.resource_url(context, 'delete') }}" method="POST" class="action"> |
||||||
|
<input type="hidden" name="csrf_token" value="{{get_csrf_token()}}"> |
||||||
|
|
||||||
|
<table class="table"> |
||||||
|
<thead> |
||||||
|
<th>Cas / Description</th> |
||||||
|
<th>Category</th> |
||||||
|
<th>Catalog Nr</th> |
||||||
|
<th>Vendor</th> |
||||||
|
<th>Package Size</th> |
||||||
|
<th>Unit Prize</th> |
||||||
|
<th>Currency</th> |
||||||
|
</thead> |
||||||
|
|
||||||
|
<tbody> |
||||||
|
{% for consumable in consumables %} |
||||||
|
<tr> |
||||||
|
<td class="column-user"> |
||||||
|
<input type="hidden" name="consumable" value="{{ consumable.id }}"> |
||||||
|
{{ consumable.cas_description }} |
||||||
|
</td> |
||||||
|
<td class="column-category">{{ consumable.category.name|capitalize }}</td> |
||||||
|
<td class="column-catalog">{{ consumable.catalog_nr }}</td> |
||||||
|
<td class="column-vendor">{{ consumable.vendor }}</td> |
||||||
|
<td class="column-pkg">{{ consumable.package_size }}</td> |
||||||
|
<td class="column-price">{{ '%.2f'|format(consumable.unit_price) }}</td> |
||||||
|
<td class="column-currency">{{ consumable.currency }}</td> |
||||||
|
</tr> |
||||||
|
{% endfor %} |
||||||
|
</tbody> |
||||||
|
</table> |
||||||
|
|
||||||
|
<fieldset class="form-actions"> |
||||||
|
<div class="right"> |
||||||
|
<button name="delete" type="submit" value="submit" class="btn btn-large btn-danger">Delete Consumable{{ 's' if consumables|length > 1 }}</button> |
||||||
|
<button name="cancel" type="submit" value="cancel" class="btn btn-large">Cancel</button> |
||||||
|
</div> |
||||||
|
</fieldset> |
||||||
|
|
||||||
|
</form> |
||||||
|
|
||||||
|
</div> |
||||||
|
</div> |
||||||
|
|
||||||
|
</div> |
||||||
|
|
||||||
|
</div> |
||||||
|
{% endblock content %} |
@ -0,0 +1,24 @@ |
|||||||
|
{% extends "ordr2:templates/layout.jinja2" %} |
||||||
|
{% import 'ordr2:templates/macros.jinja2' as macros with context %} |
||||||
|
|
||||||
|
{% block subtitle %} Admin | Consumable | {{ context.model.cas_description }} {% endblock subtitle %} |
||||||
|
|
||||||
|
{% block content %} |
||||||
|
<div class="content controls"> |
||||||
|
|
||||||
|
<div class="container-fluid"> |
||||||
|
<div class="row-fluid"> |
||||||
|
<div class="page-controls"> |
||||||
|
<h1>Edit Consumable: {{ context.model.cas_description }}</h1> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
<div class="row"> |
||||||
|
<div class="span8"> |
||||||
|
{{ macros.flash_messages() }} |
||||||
|
{{form.render()|safe}} |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
|
||||||
|
</div> |
||||||
|
{% endblock content %} |
@ -0,0 +1,72 @@ |
|||||||
|
{% extends "ordr2:templates/layout.jinja2" %} |
||||||
|
{% import 'ordr2:templates/macros.jinja2' as macros with context %} |
||||||
|
|
||||||
|
{% block subtitle %} Admin | Consumables {% endblock subtitle %} |
||||||
|
|
||||||
|
{% block content %} |
||||||
|
<div class="content controls"> |
||||||
|
<div class="container-fluid controls"> |
||||||
|
|
||||||
|
<div class="row-fluid"> |
||||||
|
<div class="span2"> |
||||||
|
<div class="page-controls"> |
||||||
|
<h1> |
||||||
|
Consumables |
||||||
|
</h1> |
||||||
|
</div> |
||||||
|
{{ macros.filter_box('Category', 'category', categories) }} |
||||||
|
</div> |
||||||
|
|
||||||
|
<div class="span10"> |
||||||
|
<div class="page-controls"> |
||||||
|
<div class="actions"> |
||||||
|
<a href="{{ request.resource_url(context, 'new') }}" rel="tooltip" data-original-title="New" class="btn-flat single"><i class="add"></i></a> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
|
||||||
|
{{ macros.flash_messages() }} |
||||||
|
|
||||||
|
{% if consumables %} |
||||||
|
<table class="table"> |
||||||
|
<thead> |
||||||
|
{{ macros.sortable_table_header('Cas / Description', 'cas') }} |
||||||
|
{{ macros.sortable_table_header('Category', 'category') }} |
||||||
|
{{ macros.sortable_table_header('Catalog Nr', 'catalog') }} |
||||||
|
{{ macros.sortable_table_header('Vendor', 'vendor') }} |
||||||
|
{{ macros.sortable_table_header('Package Size', 'pkg') }} |
||||||
|
{{ macros.sortable_table_header('Unit Prize', 'price') }} |
||||||
|
{{ macros.sortable_table_header('Currency', 'currency') }} |
||||||
|
<th>Actions</th> |
||||||
|
</thead> |
||||||
|
|
||||||
|
<tbody> |
||||||
|
{% for consumable in consumables %} |
||||||
|
<tr> |
||||||
|
<td class="column-cas">{{ consumable.model.cas_description }}</td> |
||||||
|
<td class="column-category">{{ consumable.model.category.name|capitalize }}</td> |
||||||
|
<td class="column-catalog">{{ consumable.model.catalog_nr }}</td> |
||||||
|
<td class="column-vendor">{{ consumable.model.vendor }}</td> |
||||||
|
<td class="column-pkg">{{ consumable.model.package_size }}</td> |
||||||
|
<td class="column-price">{{ '%.2f'|format(consumable.model.unit_price) }}</td> |
||||||
|
<td class="column-currency">{{ consumable.model.currency }}</td> |
||||||
|
<td> |
||||||
|
<a href="{{ request.resource_url(consumable) }}" class="action edit" title="Edit Consumable">edit</a> |
||||||
|
<a href="{{ request.resource_url(consumable, 'delete') }}" class="action delete" title="Delete Consumable">delete</a> |
||||||
|
</td> |
||||||
|
</tr> |
||||||
|
{% endfor %} |
||||||
|
</tbody> |
||||||
|
</table> |
||||||
|
|
||||||
|
{{ macros.pagination() }} |
||||||
|
{% else %} |
||||||
|
<div class="alert alert-block alert-error"> |
||||||
|
<h4 class="alert-heading">Oh snap! Nothing to display!</h4> |
||||||
|
<p>Your query didn't return any data.</p> |
||||||
|
</div> |
||||||
|
{% endif %} |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
{% endblock content %} |
@ -0,0 +1,24 @@ |
|||||||
|
{% extends "ordr2:templates/layout.jinja2" %} |
||||||
|
{% import 'ordr2:templates/macros.jinja2' as macros with context %} |
||||||
|
|
||||||
|
{% block subtitle %} Admin | Consumable | Add {% endblock subtitle %} |
||||||
|
|
||||||
|
{% block content %} |
||||||
|
<div class="content controls"> |
||||||
|
|
||||||
|
<div class="container-fluid"> |
||||||
|
<div class="row-fluid"> |
||||||
|
<div class="page-controls"> |
||||||
|
<h1>Add Consumable</h1> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
<div class="row"> |
||||||
|
<div class="span8"> |
||||||
|
{{ macros.flash_messages() }} |
||||||
|
{{form.render()|safe}} |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
</div> |
||||||
|
|
||||||
|
</div> |
||||||
|
{% endblock content %} |
Reference in new issue