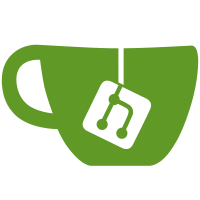
3 changed files with 74 additions and 1 deletions
@ -0,0 +1,53 @@ |
|||||||
|
import bcrypt |
||||||
|
import enum |
||||||
|
import uuid |
||||||
|
|
||||||
|
from collections import namedtuple |
||||||
|
from datetime import datetime |
||||||
|
from sqlalchemy import ( |
||||||
|
Column, |
||||||
|
Date, |
||||||
|
Enum, |
||||||
|
Float, |
||||||
|
Integer, |
||||||
|
Text, |
||||||
|
) |
||||||
|
|
||||||
|
from .meta import Base |
||||||
|
|
||||||
|
|
||||||
|
class Category(enum.Enum): |
||||||
|
''' Categories of consumables and orders ''' |
||||||
|
CHEMICAL = 'chemical' |
||||||
|
DISPOSABLE = 'disposable' |
||||||
|
SOLVENT = 'solvent' |
||||||
|
BIOLAB = 'biolab' |
||||||
|
|
||||||
|
|
||||||
|
class Consumable(Base): |
||||||
|
''' A consumable ''' |
||||||
|
|
||||||
|
__tablename__ = 'consumables' |
||||||
|
|
||||||
|
id = Column(Integer, primary_key=True) |
||||||
|
|
||||||
|
cas_description = Column(Text, nullable=False, unique=True) |
||||||
|
category = Column(Enum(Category), nullable=False) |
||||||
|
catalog_nr = Column(Text, nullable=False) |
||||||
|
vendor = Column(Text, nullable=False) |
||||||
|
package_size = Column(Text, nullable=False) |
||||||
|
unit_price = Column(Float, nullable=False) |
||||||
|
currency = Column(Text, nullable=False, default='EUR') |
||||||
|
comment = Column(Text, nullable=False, default='') |
||||||
|
date_created = Column(Date, nullable=False, default=datetime.utcnow) |
||||||
|
date_modified = Column( |
||||||
|
Date, |
||||||
|
nullable=False, |
||||||
|
default=datetime.utcnow, |
||||||
|
onupdate=datetime.utcnow |
||||||
|
) |
||||||
|
|
||||||
|
def __str__(self): |
||||||
|
''' string representation ''' |
||||||
|
return '{!s} ({!s})'.format(self.cas_description, self.vendor) |
||||||
|
|
Reference in new issue