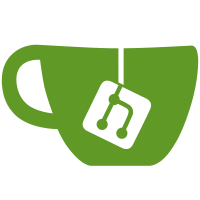
10 changed files with 416 additions and 12 deletions
@ -0,0 +1,85 @@
@@ -0,0 +1,85 @@
|
||||
def test_my_account_edit(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestUser", "jon").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/myaccount") |
||||
form = response.forms[1] |
||||
form["first_name"] = "Terry" |
||||
form["last_name"] = "Gilliam" |
||||
form["email"] = "terry@example.com" |
||||
form.submit("Save_Changes") |
||||
|
||||
login_as("TestAdmin", "jane") |
||||
|
||||
response = testapp.get("/users/", status=200) |
||||
assert "Jon" not in response |
||||
assert "Smith" not in response |
||||
assert "jon@example.com" not in response |
||||
assert "Terry" in response |
||||
assert "Gilliam" in response |
||||
assert "terry@example.com" in response |
||||
|
||||
|
||||
def test_my_account_edit_cancel(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestUser", "jon").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/myaccount") |
||||
form = response.forms[1] |
||||
form["first_name"] = "Terry" |
||||
form["last_name"] = "Gilliam" |
||||
form["email"] = "terry@example.com" |
||||
form.submit("Cancel") |
||||
|
||||
login_as("TestAdmin", "jane") |
||||
|
||||
response = testapp.get("/users/", status=200) |
||||
assert "Jon" in response |
||||
assert "Smith" in response |
||||
assert "jon@example.com" in response |
||||
assert "Terry" not in response |
||||
assert "Gilliam" not in response |
||||
assert "terry@example.com" not in response |
||||
|
||||
|
||||
def test_my_account_edit_form_error(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestUser", "jon").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/myaccount") |
||||
form = response.forms[1] |
||||
form["first_name"] = "" |
||||
form["last_name"] = "" |
||||
form["email"] = "" |
||||
response = form.submit("Save_Changes") |
||||
assert "There was a problem with your submission" in response |
||||
|
||||
|
||||
def test_my_account_reset_password(testapp, login_as, parse_latest_mail): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestUser", "jon").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/myaccount") |
||||
assert "Change Password" in response |
||||
|
||||
response = testapp.get("/mypassword").follow().follow() |
||||
assert "My Orders" in response |
||||
assert "A password reset link has been sent" in response |
||||
|
||||
parsed = parse_latest_mail() |
||||
assert "If you forgot your password" in parsed.body |
||||
|
||||
# same link target as forgotten password, rest is tested there |
||||
assert parsed.link.startswith("http://localhost/reset?t=") |
@ -0,0 +1,146 @@
@@ -0,0 +1,146 @@
|
||||
def test_user_edit(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestUser/edit") |
||||
form = response.forms[1] |
||||
form["first_name"] = "Terry" |
||||
form["last_name"] = "Gilliam" |
||||
form["email"] = "terry@example.com" |
||||
form.submit("Save_Changes") |
||||
|
||||
response = testapp.get("/users/", status=200) |
||||
assert "Jon" not in response |
||||
assert "Smith" not in response |
||||
assert "jon@example.com" not in response |
||||
assert "Terry" in response |
||||
assert "Gilliam" in response |
||||
assert "terry@example.com" in response |
||||
|
||||
|
||||
def test_user_edit_cancel(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestUser/edit") |
||||
form = response.forms[1] |
||||
form["first_name"] = "Terry" |
||||
form["last_name"] = "Gilliam" |
||||
form["email"] = "terry@example.com" |
||||
form.submit("Cancel") |
||||
|
||||
response = testapp.get("/users/", status=200) |
||||
assert "Jon" in response |
||||
assert "Smith" in response |
||||
assert "jon@example.com" in response |
||||
assert "Terry" not in response |
||||
assert "Gilliam" not in response |
||||
assert "terry@example.com" not in response |
||||
|
||||
|
||||
def test_user_edit_form_error(testapp, login_as): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestUser/edit") |
||||
form = response.forms[1] |
||||
form["first_name"] = "" |
||||
response = form.submit("Save_Changes") |
||||
assert "There was a problem with your submission" in response |
||||
|
||||
|
||||
def test_user_edit_reset_password(testapp, login_as, parse_latest_mail): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestUser/edit") |
||||
assert "Reset Password" in response |
||||
assert "Delte User" not in response |
||||
|
||||
response = testapp.get("/users/TestUser/password").follow() |
||||
assert "jon@example.com" in response |
||||
assert "A password reset link has been sent" in response |
||||
|
||||
parsed = parse_latest_mail() |
||||
assert "If you forgot your password" in parsed.body |
||||
|
||||
# same link target as forgotten password, rest is tested there |
||||
assert parsed.link.startswith("http://localhost/reset?t=") |
||||
|
||||
|
||||
def test_user_delete(testapp, login_as, parse_latest_mail): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/edit") |
||||
assert "Delete User" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/delete") |
||||
assert "I confirm that I want to delete this user." in response |
||||
|
||||
form = response.forms[1] |
||||
form["confirmation"].checked = True |
||||
form.submit("delete") |
||||
|
||||
response = testapp.get("/users") |
||||
assert "has been deleted" in response |
||||
assert "TestInactive" not in response |
||||
|
||||
|
||||
def test_user_delete_cancel(testapp, login_as, parse_latest_mail): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/edit") |
||||
assert "Delete User" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/delete") |
||||
assert "I confirm that I want to delete this user." in response |
||||
|
||||
form = response.forms[1] |
||||
form["confirmation"].checked = True |
||||
form.submit("cancel") |
||||
|
||||
response = testapp.get("/users") |
||||
assert "has been deleted" not in response |
||||
assert "TestInactive" in response |
||||
|
||||
|
||||
def test_user_delete_no_confirm(testapp, login_as, parse_latest_mail): |
||||
response = testapp.get("/", status=302).follow(status=200) |
||||
assert "Please Log In" in response |
||||
|
||||
response = login_as("TestAdmin", "jane").follow(status=200) |
||||
assert "My Orders" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/edit") |
||||
assert "Delete User" in response |
||||
|
||||
response = testapp.get("/users/TestInactive/delete") |
||||
assert "I confirm that I want to delete this user." in response |
||||
|
||||
form = response.forms[1] |
||||
form["confirmation"].checked = False |
||||
form.submit("delete") |
||||
|
||||
response = testapp.get("/users") |
||||
assert "has been deleted" not in response |
||||
assert "TestInactive" in response |
Loading…
Reference in new issue