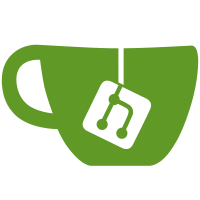
5 changed files with 104 additions and 15 deletions
@ -0,0 +1,73 @@
@@ -0,0 +1,73 @@
|
||||
import pytest |
||||
|
||||
|
||||
@pytest.fixture |
||||
def example_file(example_root): |
||||
return example_root / "Verbrauchsmittel-Toto-2020.xlsx" |
||||
|
||||
|
||||
@pytest.fixture |
||||
def example_workbook(example_file): |
||||
import openpyxl |
||||
|
||||
yield openpyxl.open(example_file) |
||||
|
||||
|
||||
@pytest.mark.parametrize( |
||||
"input,expected", |
||||
[ |
||||
("a", False), |
||||
("", True), |
||||
(" ", True), |
||||
(" a ", False), |
||||
(None, True), |
||||
(0, False), |
||||
(2.2, False), |
||||
], |
||||
) |
||||
def test_is_empty_excel_value(input, expected): |
||||
from superx_budget.helpers import is_empty_excel_value |
||||
|
||||
result = is_empty_excel_value(input) |
||||
|
||||
assert result == expected |
||||
|
||||
|
||||
@pytest.mark.parametrize( |
||||
"input,expected", |
||||
[ |
||||
("a", "a"), |
||||
("", ""), |
||||
(" ", ""), |
||||
(" a ", "a"), |
||||
(None, None), |
||||
(1, 1), |
||||
(2.2, 2.2), |
||||
], |
||||
) |
||||
def test_strip_excel_value(input, expected): |
||||
from superx_budget.helpers import strip_excel_value |
||||
|
||||
result = strip_excel_value(input) |
||||
|
||||
assert result == expected |
||||
|
||||
|
||||
def test_get_sheet_of_file_first(example_file): |
||||
from superx_budget.helpers import get_sheet_of_file |
||||
|
||||
sheet = get_sheet_of_file(example_file) # sheet=None |
||||
first_row = next(sheet.values) |
||||
first_cell = first_row[0] |
||||
|
||||
assert first_cell.strip() == "Nr." |
||||
|
||||
|
||||
def test_get_sheet_of_file_named(example_file): |
||||
from superx_budget.helpers import get_sheet_of_file |
||||
|
||||
sheet = get_sheet_of_file(example_file, sheet="Safeguard I") |
||||
first_row = next(sheet.values) |
||||
first_cell = first_row[0] |
||||
|
||||
assert first_cell == 1 |
Loading…
Reference in new issue