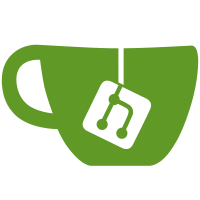
6 changed files with 274 additions and 380 deletions
@ -0,0 +1,186 @@
@@ -0,0 +1,186 @@
|
||||
from pathlib import Path |
||||
|
||||
home = Path.home() |
||||
|
||||
|
||||
import sys |
||||
from PyQt5.QtWidgets import ( |
||||
QApplication, |
||||
QMainWindow, |
||||
QPushButton, |
||||
QDesktopWidget, |
||||
QHBoxLayout, |
||||
QVBoxLayout, |
||||
QWidget, |
||||
QLabel, |
||||
QLineEdit, |
||||
QGridLayout, |
||||
QFileDialog, |
||||
) |
||||
from PyQt5.QtGui import QIcon, QIntValidator |
||||
|
||||
from .workflows import ( |
||||
prescan_workflow, |
||||
image_workflow, |
||||
data_workflow, |
||||
cached_data_workflow, |
||||
postprocessing_workflow, |
||||
) |
||||
|
||||
|
||||
class MtorImageAnalysis(QWidget): |
||||
def __init__(self): |
||||
super().__init__() |
||||
self.init_ui() |
||||
|
||||
def init_ui(self): |
||||
|
||||
# analysis parameters |
||||
self.analysis_parameters = None |
||||
|
||||
# directory browser |
||||
self.dir_label = QLabel("Image Folder") |
||||
self.dir_btn = QPushButton("Browse") |
||||
self.dir_btn.clicked.connect(self.get_tif_dir) |
||||
self.dir_selected = QLabel() |
||||
|
||||
# roi input fields |
||||
self.roi_top_label = QLabel("Top edge of ROI") |
||||
self.roi_top_input = QLineEdit() |
||||
self.roi_top_input.setValidator(QIntValidator()) |
||||
self.roi_top_input.textChanged.connect(self.check_button_state) |
||||
|
||||
self.roi_left_label = QLabel("Left edge of ROI") |
||||
self.roi_left_input = QLineEdit() |
||||
self.roi_left_input.setValidator(QIntValidator()) |
||||
self.roi_left_input.textChanged.connect(self.check_button_state) |
||||
|
||||
self.roi_right_label = QLabel("Right edge of ROI") |
||||
self.roi_right_input = QLineEdit() |
||||
self.roi_right_input.setValidator(QIntValidator()) |
||||
self.roi_right_input.textChanged.connect(self.check_button_state) |
||||
|
||||
self.roi_bottom_label = QLabel("Bottom edge of ROI") |
||||
self.roi_bottom_input = QLineEdit() |
||||
self.roi_bottom_input.setValidator(QIntValidator()) |
||||
self.roi_bottom_input.textChanged.connect(self.check_button_state) |
||||
|
||||
# action buttons |
||||
self.btn_run = QPushButton("Run", self) |
||||
self.btn_run.setEnabled(False) |
||||
self.btn_run.clicked.connect(self.run_analysis) |
||||
|
||||
self.btn_exit = QPushButton("Exit", self) |
||||
self.btn_exit.clicked.connect(QApplication.instance().quit) |
||||
|
||||
# Layout |
||||
grid = QGridLayout() |
||||
grid.setSpacing(10) |
||||
|
||||
grid.addWidget(self.dir_label, 1, 0) |
||||
grid.addWidget(self.dir_btn, 1, 1) |
||||
|
||||
grid.addWidget(self.dir_selected, 2, 0, 1, 2) |
||||
|
||||
grid.addWidget(QLabel(), 3, 0, 1, 2) |
||||
|
||||
grid.addWidget(self.roi_top_label, 4, 0) |
||||
grid.addWidget(self.roi_top_input, 4, 1) |
||||
|
||||
grid.addWidget(self.roi_right_label, 5, 0) |
||||
grid.addWidget(self.roi_right_input, 5, 1) |
||||
|
||||
grid.addWidget(self.roi_bottom_label, 6, 0) |
||||
grid.addWidget(self.roi_bottom_input, 6, 1) |
||||
|
||||
grid.addWidget(self.roi_left_label, 7, 0) |
||||
grid.addWidget(self.roi_left_input, 7, 1) |
||||
|
||||
grid.addWidget(QLabel(), 8, 0, 1, 2) |
||||
|
||||
grid.addWidget(self.btn_exit, 9, 0) |
||||
grid.addWidget(self.btn_run, 9, 1) |
||||
|
||||
self.setLayout(grid) |
||||
#self.resize(350, 300) |
||||
self.center_window_on_desktop() |
||||
self.setWindowTitle("mTor Image Analysis") |
||||
self.setWindowIcon(QIcon("web.png")) |
||||
|
||||
# helpers |
||||
self.dir_selected.setText("/Users/holgerfrey/Developer/mtor/mtor-bilder") |
||||
self.roi_top_input.setText("50") |
||||
self.roi_right_input.setText("875") |
||||
self.roi_bottom_input.setText("300") |
||||
self.roi_left_input.setText("725") |
||||
|
||||
self.show() |
||||
|
||||
def center_window_on_desktop(self): |
||||
cp = QDesktopWidget().availableGeometry().center() |
||||
fg = self.frameGeometry() |
||||
fg.moveCenter(cp) |
||||
self.move(fg.topLeft()) |
||||
|
||||
def get_tif_dir(self): |
||||
dlg = QFileDialog(directory=str(home)) |
||||
dlg.setFileMode(QFileDialog.Directory) |
||||
|
||||
if dlg.exec_(): |
||||
filenames = dlg.selectedFiles() |
||||
folder = Path(filenames[0]) |
||||
items = (i for i in folder.iterdir() if not i.stem.startswith(".")) |
||||
tifs = [i for i in items if i.suffix == ".tif"] |
||||
if len(tifs) == 0: |
||||
self.dir_selected.setText("") |
||||
self.check_button_state() |
||||
else: |
||||
self.dir_selected.setText(filenames[0]) |
||||
self.check_button_state() |
||||
|
||||
|
||||
def check_button_state(self): |
||||
values = self.get_values() |
||||
self.btn_run.setEnabled(all(values)) |
||||
|
||||
def get_values(self): |
||||
fields = [ |
||||
(self.dir_selected, str), |
||||
(self.roi_top_input, int), |
||||
(self.roi_right_input, int), |
||||
(self.roi_bottom_input, int), |
||||
(self.roi_left_input, int), |
||||
] |
||||
result = [] |
||||
for field, func in fields: |
||||
raw_data = field.text().strip() |
||||
if raw_data: |
||||
if func is int: |
||||
# in qt5, a point as 1000 separator is allowed |
||||
raw_data = raw_data.replace(".", "") |
||||
result.append(func(raw_data)) |
||||
else: |
||||
result.append(None) |
||||
return tuple(result) |
||||
|
||||
def run_analysis(self): |
||||
parameters = self.get_values() |
||||
if all(parameters): |
||||
self.analysis_parameters = parameters |
||||
self.hide() |
||||
QApplication.instance().quit() |
||||
|
||||
|
||||
def run_gui(): |
||||
app = QApplication(sys.argv) |
||||
mia = MtorImageAnalysis() |
||||
app.exec_() |
||||
|
||||
if mia.analysis_parameters is not None: |
||||
analysis_parameters = tuple( (p for p in mia.analysis_parameters) ) |
||||
pw = prescan_workflow(*analysis_parameters) |
||||
iw = image_workflow(pw.parameters) |
||||
dw = data_workflow(iw.data, iw.parameters) |
||||
fw = postprocessing_workflow(dw.data, dw.parameters) # noqa: F841 |
||||
|
||||
sys.exit() |
Loading…
Reference in new issue