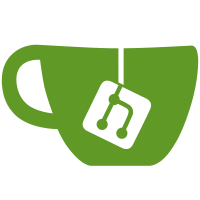
4 changed files with 106 additions and 1 deletions
@ -0,0 +1,11 @@
@@ -0,0 +1,11 @@
|
||||
[[source]] |
||||
url = "https://pypi.org/simple" |
||||
verify_ssl = true |
||||
name = "pypi" |
||||
|
||||
[packages] |
||||
|
||||
[dev-packages] |
||||
|
||||
[requires] |
||||
python_version = "3.7" |
@ -1,3 +1,33 @@
@@ -1,3 +1,33 @@
|
||||
# pysendmail |
||||
|
||||
Simple module for using sendmail from Python. |
||||
Simple tool for sending email with sendmail, postfix or ssmtpd. |
||||
|
||||
Example: |
||||
{{{ |
||||
import pysema |
||||
|
||||
pysema.send( |
||||
['alice@example.com', 'bob@example.com'], |
||||
'really good subject line', |
||||
'Message body goes here' |
||||
) |
||||
}}} |
||||
|
||||
If you need to send some complex message, use [email.message][em] from the |
||||
Python standard library to construct the message (including header fields) and |
||||
pass it to the `send_message` function: |
||||
|
||||
{{{ |
||||
import pysema |
||||
from email.message import EmailMessage |
||||
|
||||
msg = EmailMessage() |
||||
# compose message |
||||
|
||||
pysema.send_message(msg) |
||||
}}} |
||||
|
||||
if something goes whoopsie a `pysema.SendMailException` is raised. |
||||
|
||||
|
||||
[em]: https://docs.python.org/3/library/email.message.html |
@ -0,0 +1,63 @@
@@ -0,0 +1,63 @@
|
||||
''' simple tool for sending email with sendmail, postfix or ssmtpd |
||||
|
||||
import pysema |
||||
send( |
||||
['alice@example.com', 'bob@example.com'], |
||||
'really good subject line', |
||||
'Message body goes here' |
||||
) |
||||
''' |
||||
|
||||
import subprocess |
||||
from email.message import EmailMessage |
||||
|
||||
|
||||
SENDMAIL_PATH = '/usr/bin/sendmail' |
||||
|
||||
|
||||
class SendMailException(Exception): |
||||
''' Exception while using pysema ''' |
||||
pass |
||||
|
||||
|
||||
def send(to=None, subject='', message='', **headers): |
||||
msg = create_message(to, subject, message, **headers) |
||||
send_message(msg) |
||||
|
||||
|
||||
def create_message(to=None, subject='', message='', **headers): |
||||
if isinstance(to, str): |
||||
recipients = to |
||||
elif isinstance(to, (list, set, tuple)): |
||||
recipients = ', '.join(to) |
||||
else: |
||||
raise SendMailException('No recipients given') |
||||
|
||||
# ensure that the 'from' keyword (if given) is uppercase |
||||
sender_mail = headers.pop('from', None) |
||||
if sender_mail is not None: |
||||
headers['From'] = sender_mail |
||||
|
||||
headers['To'] = recipients |
||||
headers['Subject'] = subject |
||||
|
||||
msg = EmailMessage() |
||||
msg.set_content(message) |
||||
for key, value in headers.items(): |
||||
msg[key] = value |
||||
|
||||
return msg |
||||
|
||||
|
||||
def send_message(msg): |
||||
if not isinstance(msg, EmailMessage): |
||||
raise SendMailException('Type Error: not an EmailMessage') |
||||
|
||||
try: |
||||
subprocess.run( |
||||
[SENDMAIL_PATH, '-t', '-oi'], |
||||
input=msg.as_bytes(), |
||||
check=True |
||||
) |
||||
except subprocess.CalledProcessError as e: |
||||
raise SendMailException from e |
Loading…
Reference in new issue