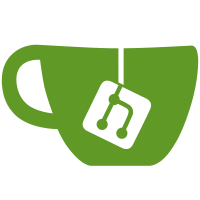
5 changed files with 72 additions and 62 deletions
@ -0,0 +1,42 @@
@@ -0,0 +1,42 @@
|
||||
''' functional tests for ordr2.views.pages ''' |
||||
|
||||
import pytest |
||||
|
||||
from . import testappsetup, testapp # noqa: F401 |
||||
|
||||
|
||||
def test_login_get(testapp): # noqa: F811 |
||||
result = testapp.get('/login') |
||||
active = result.html.find('li', class_='active') |
||||
assert active.a['href'] == '/' |
||||
expected = {'/', '/faq', '/register', '/forgot', '/register'} |
||||
hrefs = {a['href'] for a in result.html.find_all('a')} |
||||
assert expected == hrefs |
||||
forms = result.html.find_all('form') |
||||
assert len(forms) == 1 |
||||
login_form = forms[0] |
||||
assert login_form['action'] == '/login' |
||||
assert login_form['method'] == 'POST' |
||||
assert 'account is not activated' not in result |
||||
|
||||
|
||||
def test_login_ok(testapp): # noqa: F811 |
||||
result = testapp.get('/login') |
||||
login_form = result.forms[0] |
||||
login_form['username'] = 'TerryGilliam' |
||||
login_form['password'] = 'Terry' |
||||
result = login_form.submit() |
||||
assert result.location == 'http://localhost/' |
||||
|
||||
|
||||
@pytest.mark.parametrize( # noqa: F811 |
||||
'username,password', |
||||
[('John', 'Cleese'), ('unknown user', 'wrong password')] |
||||
) |
||||
def test_login_denied(testapp, username, password): |
||||
result = testapp.get('/login') |
||||
login_form = result.forms[0] |
||||
login_form['username'] = 'John' |
||||
login_form['password'] = 'Cleese' |
||||
result = login_form.submit() |
||||
assert 'account is not activated' in result |
Reference in new issue