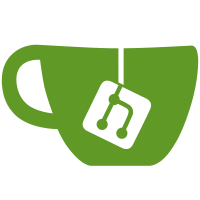
2 changed files with 51 additions and 1 deletions
@ -0,0 +1,50 @@ |
|||||||
|
from pyramid.authentication import AuthTktAuthenticationPolicy |
||||||
|
from pyramid.authorization import ACLAuthorizationPolicy |
||||||
|
from pyramid.security import Authenticated, Everyone |
||||||
|
|
||||||
|
from .models import User |
||||||
|
|
||||||
|
|
||||||
|
class AuthenticationPolicy(AuthTktAuthenticationPolicy): |
||||||
|
''' How to authenticate users ''' |
||||||
|
|
||||||
|
def authenticated_userid(self, request): |
||||||
|
''' returns the id of an authenticated user |
||||||
|
|
||||||
|
heavy lifting done in get_user() attached to request |
||||||
|
''' |
||||||
|
user = request.user |
||||||
|
if user is not None: |
||||||
|
return user.id |
||||||
|
|
||||||
|
def effective_principals(self, request): |
||||||
|
''' returns a list of principals for the user ''' |
||||||
|
principals = [Everyone] |
||||||
|
user = request.user |
||||||
|
if user is not None: |
||||||
|
principals.append(Authenticated) |
||||||
|
principals.append(user.principal) |
||||||
|
principals.extend(user.role_principals) |
||||||
|
return principals |
||||||
|
|
||||||
|
|
||||||
|
def get_user(request): |
||||||
|
''' retrieves the user object by the unauthenticated user id ''' |
||||||
|
user_id = request.unauthenticated_userid |
||||||
|
if user_id is not None: |
||||||
|
user = request.dbsession.query(User).filter_by(id=user_id).first() |
||||||
|
if user and user.is_active: |
||||||
|
return user |
||||||
|
return None |
||||||
|
|
||||||
|
|
||||||
|
def includeme(config): |
||||||
|
''' initializing authentication and authorization for the Pyramid app ''' |
||||||
|
settings = config.get_settings() |
||||||
|
authn_policy = AuthenticationPolicy( |
||||||
|
settings['auth.secret'], |
||||||
|
hashalg='sha512', |
||||||
|
) |
||||||
|
config.set_authentication_policy(authn_policy) |
||||||
|
config.set_authorization_policy(ACLAuthorizationPolicy()) |
||||||
|
config.add_request_method(get_user, 'user', reify=True) |
Reference in new issue