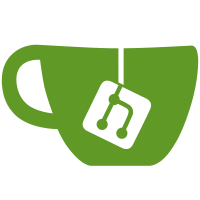
3 changed files with 101 additions and 56 deletions
@ -0,0 +1,35 @@ |
|||||||
|
''' Resources for account registraion and settings ''' |
||||||
|
|
||||||
|
from pyramid.security import Allow, Authenticated, Everyone, Deny, DENY_ALL |
||||||
|
|
||||||
|
from ordr2.resources.base import BaseResource |
||||||
|
|
||||||
|
|
||||||
|
class AccountResource(BaseResource): |
||||||
|
''' Resouce class for account registration and settings ''' |
||||||
|
|
||||||
|
#: name of the main navigation section for template highlighting |
||||||
|
nav_section = 'account' |
||||||
|
|
||||||
|
def __init__(self, name, parent, model=None): |
||||||
|
''' Create a base resource ''' |
||||||
|
super().__init__(name, parent) |
||||||
|
# the current model depends is the current logged in user or None |
||||||
|
self.model = self.request.user |
||||||
|
|
||||||
|
def __acl__(self): |
||||||
|
''' access controll list for the resource |
||||||
|
|
||||||
|
- everyone can log in our log out |
||||||
|
- authenticated users can change their settings |
||||||
|
- unauthenticated users can register |
||||||
|
|
||||||
|
''' |
||||||
|
return [ |
||||||
|
(Allow, Everyone, 'login'), |
||||||
|
(Allow, Everyone, 'logout'), |
||||||
|
(Deny, Authenticated, 'register'), |
||||||
|
(Allow, Everyone, 'register'), |
||||||
|
(Allow, Authenticated, 'settings'), |
||||||
|
] |
||||||
|
|
@ -0,0 +1,58 @@ |
|||||||
|
''' Base resource classes ''' |
||||||
|
|
||||||
|
|
||||||
|
class BaseResource(object): |
||||||
|
''' |
||||||
|
Base resouce class for location aware resources |
||||||
|
|
||||||
|
:param str name: |
||||||
|
url path segment that identifies this resource in its lineage |
||||||
|
:param parent: |
||||||
|
parent resource |
||||||
|
:type parent: |
||||||
|
ordr2.resources.BaseResource |
||||||
|
:param model: |
||||||
|
(optional) a model instance represented by this resource, |
||||||
|
e.g. a database entry |
||||||
|
|
||||||
|
Provides a dict like interface for retrieving child resources used by |
||||||
|
traversal style routing in the Pyramid web framework. |
||||||
|
|
||||||
|
The ``nodes`` property is a dictionary to to match the next url path |
||||||
|
segment in traversal to a child class. |
||||||
|
For example: to return an AccountResouce when the next path segment is |
||||||
|
'account' use ``nodes = {'account': AccountResource}``. |
||||||
|
|
||||||
|
''' |
||||||
|
|
||||||
|
# __name__ and __parent__ properties for location aware resources |
||||||
|
__name__ = None |
||||||
|
__parent__ = None |
||||||
|
|
||||||
|
#: name of the main navigation section for template highlighting |
||||||
|
nav_section = None |
||||||
|
|
||||||
|
#: dict to match the next url path segment |
||||||
|
nodes = {} |
||||||
|
|
||||||
|
def __init__(self, name, parent, model=None): |
||||||
|
''' Create a base resource ''' |
||||||
|
self.__name__ = name |
||||||
|
self.__parent__ = parent |
||||||
|
self.request = parent.request #: the current request |
||||||
|
self.model = model #: a related model instance |
||||||
|
|
||||||
|
def __acl__(self): |
||||||
|
''' access controll list for the resource ''' |
||||||
|
return [DENY_ALL] |
||||||
|
|
||||||
|
def __getitem__(self, key): |
||||||
|
''' provides the dict like interface to access child resources |
||||||
|
|
||||||
|
:param str key: |
||||||
|
path segment for a child resource |
||||||
|
:rtype: |
||||||
|
ordr2.resources.BaseResource or KeyError |
||||||
|
''' |
||||||
|
node_class = self.nodes[key] |
||||||
|
return node_class(key, self) |
Reference in new issue