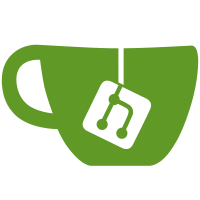
5 changed files with 92 additions and 22 deletions
@ -1,18 +0,0 @@
@@ -1,18 +0,0 @@
|
||||
from sqlalchemy import ( |
||||
Column, |
||||
Index, |
||||
Integer, |
||||
Text, |
||||
) |
||||
|
||||
from .meta import Base |
||||
|
||||
|
||||
class MyModel(Base): |
||||
__tablename__ = 'models' |
||||
id = Column(Integer, primary_key=True) |
||||
name = Column(Text) |
||||
value = Column(Integer) |
||||
|
||||
|
||||
Index('my_index', MyModel.name, unique=True, mysql_length=255) |
@ -0,0 +1,81 @@
@@ -0,0 +1,81 @@
|
||||
import bcrypt |
||||
import enum |
||||
|
||||
from collections import namedtuple |
||||
from datetime import datetime |
||||
from sqlalchemy import ( |
||||
Column, |
||||
Date, |
||||
Enum, |
||||
Integer, |
||||
Text, |
||||
) |
||||
|
||||
from .meta import Base |
||||
|
||||
|
||||
class Role(enum.Enum): |
||||
''' roles of the user ''' |
||||
NEW = 'new' |
||||
USER = 'user' |
||||
PURCHASER = 'purchaser' |
||||
ADMIN = 'admin' |
||||
INACTIVE = 'inactive' |
||||
|
||||
@property |
||||
def principal(self): |
||||
return 'role:' + self.value |
||||
|
||||
|
||||
class User(Base): |
||||
''' A user of the application ''' |
||||
|
||||
__tablename__ = 'users' |
||||
|
||||
id = Column(Integer, primary_key=True) |
||||
|
||||
user_name = Column(Text, nullable=False, unique=True) |
||||
first_name = Column(Text, nullable=False) |
||||
last_name = Column(Text, nullable=False) |
||||
email = Column(Text, nullable=False, unique=True) |
||||
password_hash = Column(Text, nullable=False) |
||||
role = Column(Enum(Role), nullable=False) |
||||
date_created = Column(Date, nullable=False, default=datetime.utcnow) |
||||
|
||||
@property |
||||
def principal(self): |
||||
''' returns the principal identifier for the user ''' |
||||
return 'user:' + str(self.id) |
||||
|
||||
@property |
||||
def role_principals(self): |
||||
''' returns the principal identifiers for the user's role ''' |
||||
principals = [self.role.principal] |
||||
if self.role is Role.PURCHASER: |
||||
principals.append(Role.USER.principal) |
||||
elif self.role is Role.ADMIN: |
||||
principals.append(Role.USER.principal) |
||||
principals.append(Role.PURCHASER.principal) |
||||
return principals |
||||
|
||||
@property |
||||
def is_active(self): |
||||
''' check if it is an active user account ''' |
||||
return self.role in (Role.USER, Role.PURCHASER, Role.ADMIN) |
||||
|
||||
def set_password(self, password): |
||||
''' hashes a new password ''' |
||||
pwhash = bcrypt.hashpw(password.encode('utf8'), bcrypt.gensalt()) |
||||
self.password_hash = pwhash.decode('utf8') |
||||
|
||||
def check_password(self, password): |
||||
''' compares a password with a stored password hash ''' |
||||
if self.password_hash: |
||||
expected_hash = self.password_hash.encode('utf8') |
||||
return bcrypt.checkpw(password.encode('utf8'), expected_hash) |
||||
return False |
||||
|
||||
def __str__(self): |
||||
''' string representation ''' |
||||
return '{!s}'.format(self.user_name) |
||||
|
Reference in new issue