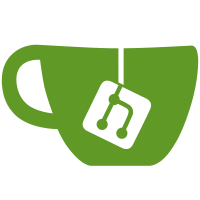
2 changed files with 120 additions and 2 deletions
@ -1 +1,118 @@
@@ -1 +1,118 @@
|
||||
$(document).ready(function() {
function capitalize(s){
return s.replace( /\b./g, function(a){ return a.toUpperCase(); } );
};
function generate_user_name() {
var first_name = $('.registration .item-first_name input').val();
var last_name = $('.registration .item-last_name input').val();
var user_name = capitalize(first_name) + capitalize(last_name);
return user_name.replace( /\s/g, '')
};
// autocomplete of the username (registration form)
$('.registration .item-user_name input').val( generate_user_name() );
$('.registration .item-first_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration .item-last_name input').keyup(function() {
$('.registration .item-user_name input').val( generate_user_name() );
});
$('.registration #deformCreate_Account').click(function() {
$('.registration .item-user_name input').removeAttr('disabled');
return true;
});
// "dispatch" clicking a th to the corresponding anchor
$('th.sortable').click( function() {
window.location = $(this).children('a').attr('href');
});
// "dispatch" clicking list item in collapse to the corresponding anchor
$('.accordion li').click( function() {
window.location = $(this).children('a').attr('href');
});
// the mark all
$('input[name="mark_all"]').click(function() {
var checked_status = this.checked;
$('input[name*="marked"]').each(function() {
this.checked = checked_status;
});
if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':hidden') ) {
$('.marking-needed').fadeIn("fast");
} else if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':visible') ) {
// do nothing
} else {
$('.marking-needed').fadeOut("fast");
}
});
// show actions only if some data is marked
$('input[name*="marked"]').change(function() {
if( $('input[name*="marked"]').is(':checked') ) {
if( $('.marking-needed').is(':hidden') )
$('.marking-needed').fadeIn("slow");
} else {
$('.marking-needed').fadeOut("slow");
}
});
// quick-actions
$('.quick-action a[data-value]').click(function(event) {
event.preventDefault();
var value = $(this).attr('data-value');
var action = $(this).closest('div').attr('data-action');
$('select[name*='+action+']').each(function() {
$(this).val(value);
});
});
// submit search
$('.search-form input[type="search"]').keypress(function(event) {
if( event.keyCode == 13 && $('.typeahead.dropdown-menu').is(':hidden') )
$('.search-form').submit();
});
// aside filter
$('.filter input[type="checkbox"]').click( function() {
if( $(this).is(':checked') ) {
$(this).parents('li').addClass('active');
} else {
$(this).parents('li').removeClass('active');
}
});
// tooltips
$('.page-controls').tooltip({
selector: "[rel=tooltip]"
});
// calculator
if ( $('input[name="price_unit"]').length ) {
if( $('input[name="price_unit"]').val() != '' && $('input[name="quantity"]').val() != '' ){
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
}
$('input[name="price_unit"], input[name="quantity"]').keyup(function() {
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val();
total = Math.round(total*100)/100;
$('input[name="price_total_disabled"]').attr( 'placeholder', total );
});
}
if ( $('input[name="currency"]').length ) {
// added currency to total price
if( $('input[name="currency"]').val() != '' ){
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
}
$('input[name="currency"]').keyup(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
$('input[name="currency"]').change(function() {
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() );
});
}
}); |
||||
$(document).ready(function() { |
||||
|
||||
function capitalize(s){ |
||||
return s.replace( /\b./g, function(a){ return a.toUpperCase(); } ); |
||||
}; |
||||
|
||||
function generate_user_name() { |
||||
var first_name = $('.registration .item-first_name input').val(); |
||||
var last_name = $('.registration .item-last_name input').val(); |
||||
var user_name = capitalize(first_name) + capitalize(last_name); |
||||
return user_name.replace( /\s/g, '') |
||||
}; |
||||
|
||||
// autocomplete of the username (registration form)
|
||||
|
||||
//$('.registration .item-user_name input').val( generate_user_name() );
|
||||
$('.registration .item-first_name input').keyup(function() { |
||||
$('.registration .item-user_name input').val( generate_user_name() ); |
||||
}); |
||||
$('.registration .item-last_name input').keyup(function() { |
||||
$('.registration .item-user_name input').val( generate_user_name() ); |
||||
}); |
||||
|
||||
|
||||
|
||||
// "dispatch" clicking a th to the corresponding anchor
|
||||
$('th.sortable').click( function() { |
||||
window.location = $(this).children('a').attr('href'); |
||||
}); |
||||
|
||||
// "dispatch" clicking list item in collapse to the corresponding anchor
|
||||
$('.accordion li').click( function() { |
||||
window.location = $(this).children('a').attr('href'); |
||||
}); |
||||
|
||||
// the mark all
|
||||
$('input[name="mark_all"]').click(function() { |
||||
var checked_status = this.checked; |
||||
$('input[name*="marked"]').each(function() { |
||||
this.checked = checked_status; |
||||
}); |
||||
if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':hidden') ) { |
||||
$('.marking-needed').fadeIn("fast"); |
||||
} else if( $('input[name="mark_all"]').is(':checked') && $('.marking-needed').is(':visible') ) { |
||||
// do nothing
|
||||
} else { |
||||
$('.marking-needed').fadeOut("fast"); |
||||
} |
||||
}); |
||||
|
||||
// show actions only if some data is marked
|
||||
$('input[name="marked"]').change(function() { |
||||
if( $('input[name*="marked"]').is(':checked') ) { |
||||
if( $('.marking-needed').is(':hidden') ) |
||||
$('.marking-needed').fadeIn("slow"); |
||||
} else { |
||||
$('.marking-needed').fadeOut("slow"); |
||||
} |
||||
}); |
||||
|
||||
// quick-actions
|
||||
$('.quick-action a[data-value]').click(function(event) { |
||||
event.preventDefault(); |
||||
var value = $(this).attr('data-value'); |
||||
var action = $(this).closest('div').attr('data-action'); |
||||
$('select[name*='+action+']').each(function() { |
||||
$(this).val(value); |
||||
}); |
||||
}); |
||||
|
||||
// submit search
|
||||
$('.search-form input[type="search"]').keypress(function(event) { |
||||
if( event.keyCode == 13 && $('.typeahead.dropdown-menu').is(':hidden') ) |
||||
$('.search-form').submit(); |
||||
}); |
||||
|
||||
// aside filter
|
||||
$('.filter input[type="checkbox"]').click( function() { |
||||
if( $(this).is(':checked') ) { |
||||
$(this).parents('li').addClass('active'); |
||||
} else { |
||||
$(this).parents('li').removeClass('active'); |
||||
} |
||||
}); |
||||
|
||||
// tooltips
|
||||
$('.page-controls').tooltip({ |
||||
selector: "[rel=tooltip]" |
||||
}); |
||||
|
||||
// calculator
|
||||
if ( $('input[name="price_unit"]').length ) { |
||||
if( $('input[name="price_unit"]').val() != '' && $('input[name="quantity"]').val() != '' ){ |
||||
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val(); |
||||
total = Math.round(total*100)/100; |
||||
$('input[name="price_total_disabled"]').attr( 'placeholder', total ); |
||||
} |
||||
$('input[name="price_unit"], input[name="quantity"]').keyup(function() { |
||||
var total = $('input[name="price_unit"]').val().replace(",", ".") * $('input[name="quantity"]').val(); |
||||
total = Math.round(total*100)/100; |
||||
$('input[name="price_total_disabled"]').attr( 'placeholder', total ); |
||||
}); |
||||
} |
||||
|
||||
if ( $('input[name="currency"]').length ) { |
||||
// added currency to total price
|
||||
if( $('input[name="currency"]').val() != '' ){ |
||||
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() ); |
||||
} |
||||
$('input[name="currency"]').keyup(function() { |
||||
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() ); |
||||
}); |
||||
$('input[name="currency"]').change(function() { |
||||
$('input[name="currency_disabled"]').attr( 'placeholder', $('input[name="currency"]').val() ); |
||||
}); |
||||
} |
||||
|
||||
}); |
||||
|
Reference in new issue