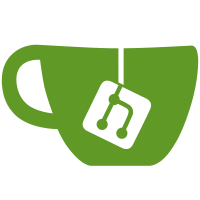
5 changed files with 100 additions and 14 deletions
@ -0,0 +1,29 @@
@@ -0,0 +1,29 @@
|
||||
''' Resources (sub) package, used to connect URLs to views ''' |
||||
|
||||
from pyramid.security import Allow, Everyone, DENY_ALL |
||||
|
||||
|
||||
class RegistrationResource: |
||||
''' The resource for new user registration |
||||
|
||||
:param pyramid.request.Request request: the current request object |
||||
:param str name: the name of the resource |
||||
:param parent: the parent resouce |
||||
''' |
||||
|
||||
nav_active = 'welcome' |
||||
|
||||
def __init__(self, request, name, parent): |
||||
''' Create registration resource |
||||
|
||||
:param pyramid.request.Request request: the current request object |
||||
:param str name: the name of the resource |
||||
:param parent: the parent resouce |
||||
''' |
||||
self.request = request |
||||
self.__name__ = name |
||||
self.__parent__ = parent |
||||
|
||||
def __acl__(self): |
||||
''' access controll list for the resource ''' |
||||
return [(Allow, Everyone, 'view'), DENY_ALL] |
@ -0,0 +1,20 @@
@@ -0,0 +1,20 @@
|
||||
''' Tests for the account resources ''' |
||||
|
||||
|
||||
def test_registration_init(): |
||||
from ordr.resources.account import RegistrationResource |
||||
resource = RegistrationResource( |
||||
request='some request', |
||||
name='a name', |
||||
parent='the parent' |
||||
) |
||||
assert resource.__name__ == 'a name' |
||||
assert resource.__parent__ == 'the parent' |
||||
assert resource.request == 'some request' |
||||
|
||||
|
||||
def test_registration_acl(): |
||||
from pyramid.security import Allow, Everyone, DENY_ALL |
||||
from ordr.resources.account import RegistrationResource |
||||
resource = RegistrationResource('some request', 'a name', 'the parent') |
||||
assert resource.__acl__() == [(Allow, Everyone, 'view'), DENY_ALL] |
@ -0,0 +1,36 @@
@@ -0,0 +1,36 @@
|
||||
''' Tests for the root resource ''' |
||||
|
||||
import pytest |
||||
|
||||
|
||||
def test_root_init(): |
||||
from ordr.resources import RootResource |
||||
root = RootResource('request') |
||||
assert root.__name__ is None |
||||
assert root.__parent__ is None |
||||
assert root.request == 'request' |
||||
|
||||
|
||||
def test_root_acl(): |
||||
from pyramid.security import Allow, Everyone, DENY_ALL |
||||
from ordr.resources import RootResource |
||||
root = RootResource(None) |
||||
assert root.__acl__() == [(Allow, Everyone, 'view'), DENY_ALL] |
||||
|
||||
|
||||
def test_root_getitem(): |
||||
from ordr.resources import RootResource |
||||
from ordr.resources.account import RegistrationResource |
||||
root = RootResource(None) |
||||
child = root['registration'] |
||||
assert isinstance(child, RegistrationResource) |
||||
assert child.__name__ == 'registration' |
||||
assert child.__parent__ == root |
||||
assert child.request == root.request |
||||
|
||||
|
||||
def test_root_getitem_raises_error(): |
||||
from ordr.resources import RootResource |
||||
root = RootResource(None) |
||||
with pytest.raises(KeyError): |
||||
root['unknown child name'] |
@ -1,14 +0,0 @@
@@ -1,14 +0,0 @@
|
||||
|
||||
def test_root_init(): |
||||
from ordr.resources import RootResource |
||||
root = RootResource('request') |
||||
assert root.__name__ is None |
||||
assert root.__parent__ is None |
||||
assert root.request == 'request' |
||||
|
||||
|
||||
def test_root_acl(): |
||||
from pyramid.security import Allow, Everyone, DENY_ALL |
||||
from ordr.resources import RootResource |
||||
root = RootResource(None) |
||||
assert root.__acl__() == [(Allow, Everyone, 'view'), DENY_ALL] |
Reference in new issue