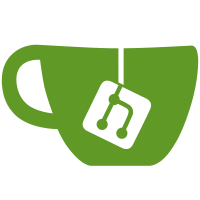
9 changed files with 209 additions and 52 deletions
@ -1,3 +1,64 @@ |
|||||||
# -*- coding: utf-8 -*- |
# -*- coding: utf-8 -*- |
||||||
|
|
||||||
"""Main module.""" |
"""Main module.""" |
||||||
|
|
||||||
|
import re |
||||||
|
|
||||||
|
from collections import namedtuple |
||||||
|
from pathlib import Path |
||||||
|
|
||||||
|
REGEX_ID = r"(?P<row>[A-H])(?P<col>(0?[1-9]|1[0-2]))_(?P<channel>\d)" |
||||||
|
REGEX_SENSOVATION = r"(?P<stem>(.+_|))" + REGEX_ID |
||||||
|
REGEX_SCIENION = REGEX_ID + r"(?P<stem>(.+_|))" |
||||||
|
|
||||||
|
RE_SENSOVATION = re.compile(REGEX_SENSOVATION) |
||||||
|
RE_SCIENION = re.compile(REGEX_SENSOVATION) |
||||||
|
|
||||||
|
|
||||||
|
TPL_SENSOVATION = '{r.stem}_{r.row}{r.col:0>{dec}d}_{r.channel}' |
||||||
|
|
||||||
|
class S2IOError(IOError): |
||||||
|
""" custom IO Exception """ |
||||||
|
|
||||||
|
|
||||||
|
Renamable = namedtuple("Renamable", "path,stem,row,col,channel") |
||||||
|
|
||||||
|
|
||||||
|
def get_source_list(folder, suffix=None): |
||||||
|
""" returns the files in a directory that are not hidden |
||||||
|
|
||||||
|
if 'suffix' is given, the list will only return files with the given |
||||||
|
extension |
||||||
|
""" |
||||||
|
src_dir = Path(folder) |
||||||
|
if not src_dir.is_dir(): |
||||||
|
raise S2IOError(f"{src_dir} is not a directory") |
||||||
|
all_files = (f for f in src_dir.iterdir() if f.is_file()) |
||||||
|
non_hidden_files = (f for f in all_files if not f.stem.startswith(".")) |
||||||
|
if suffix is None: |
||||||
|
return non_hidden_files |
||||||
|
else: |
||||||
|
return filter_source_list(non_hidden_files, suffix) |
||||||
|
|
||||||
|
|
||||||
|
def filter_source_list(source_list, suffix): |
||||||
|
""" filters pathlib.Path objects by only containing a given suffix """ |
||||||
|
return (f for f in source_list if f.suffix.lower() == suffix) |
||||||
|
|
||||||
|
|
||||||
|
def parse_source_list(source_list, regex): |
||||||
|
""" returns a list of items that can be renamed """ |
||||||
|
for path in source_list: |
||||||
|
groups = regex.match(path.stem) |
||||||
|
if groups: |
||||||
|
stem = groups["stem"] |
||||||
|
if stem.endswith('_'): |
||||||
|
stem = stem[:-1] |
||||||
|
entry = Renamable( |
||||||
|
path=path, |
||||||
|
stem=stem, |
||||||
|
row=groups["row"], |
||||||
|
col=int(groups["col"]), |
||||||
|
channel=groups["channel"], |
||||||
|
) |
||||||
|
yield entry |
||||||
|
@ -0,0 +1,21 @@ |
|||||||
|
#!/usr/bin/env python |
||||||
|
# -*- coding: utf-8 -*- |
||||||
|
|
||||||
|
"""Tests for `s2rename` package.""" |
||||||
|
|
||||||
|
import pytest |
||||||
|
|
||||||
|
from click.testing import CliRunner |
||||||
|
|
||||||
|
from s2rename import cli |
||||||
|
|
||||||
|
|
||||||
|
def test_command_line_interface(): |
||||||
|
"""Test the CLI.""" |
||||||
|
runner = CliRunner() |
||||||
|
result = runner.invoke(cli.main) |
||||||
|
assert result.exit_code == 0 |
||||||
|
assert "s2rename.cli.main" in result.output |
||||||
|
help_result = runner.invoke(cli.main, ["--help"]) |
||||||
|
assert help_result.exit_code == 0 |
||||||
|
assert "--help Show this message and exit." in help_result.output |
Reference in new issue