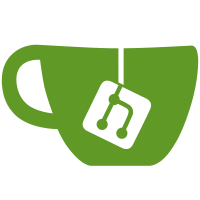
6 changed files with 264 additions and 146 deletions
@ -1,21 +0,0 @@
@@ -1,21 +0,0 @@
|
||||
#!/usr/bin/env python |
||||
# -*- coding: utf-8 -*- |
||||
|
||||
"""Tests for `s2rename` package.""" |
||||
|
||||
import pytest |
||||
|
||||
from click.testing import CliRunner |
||||
|
||||
from s2rename import cli |
||||
|
||||
|
||||
def test_command_line_interface(): |
||||
"""Test the CLI.""" |
||||
runner = CliRunner() |
||||
result = runner.invoke(cli.main) |
||||
assert result.exit_code == 0 |
||||
assert "s2rename.cli.main" in result.output |
||||
help_result = runner.invoke(cli.main, ["--help"]) |
||||
assert help_result.exit_code == 0 |
||||
assert "--help Show this message and exit." in help_result.output |
@ -0,0 +1,182 @@
@@ -0,0 +1,182 @@
|
||||
#!/usr/bin/env python |
||||
# -*- coding: utf-8 -*- |
||||
|
||||
"""Tests for `s2rename` package.""" |
||||
|
||||
import pytest |
||||
|
||||
from pathlib import Path |
||||
|
||||
|
||||
sensovation_source_list = [ |
||||
Path("some thing_A1_1.tif"), |
||||
Path("some thing_A02_2.tif"), |
||||
Path("A03_3.tif"), |
||||
Path("some thing_A10_4"), |
||||
Path("some thing_A11_5.csv"), |
||||
Path("some thing_A12_6.xml"), |
||||
Path("some thing_B01_1.tif"), |
||||
Path("some thing_C01_1.tif"), |
||||
Path("some thing_D01_1.tif"), |
||||
Path("some thing_E01_1.tif"), |
||||
Path("some thing_F01_1.tif"), |
||||
Path("some thing_G01_1.tif"), |
||||
Path("some thing_H01_1.tif"), |
||||
Path("some thing_I01_1.tif"), |
||||
Path("some thing_FF01_1.tif"), |
||||
Path("some thing_F21_1.tif"), |
||||
Path("some thing_F00_1.tif"), |
||||
Path("some thing_F0_1.tif"), |
||||
Path("some thing_F13_1.tif"), |
||||
Path("some thing_F08.tif"), |
||||
Path(".some thing_F09_1.tif"), |
||||
] |
||||
|
||||
scienion_source_list = [ |
||||
Path("A1_1_some thing.tif"), |
||||
Path("A02_2_some thing.tif"), |
||||
Path("A03_3.tif"), |
||||
Path("A10_4_some thing"), |
||||
Path("A11_5_some thing.csv"), |
||||
Path("A12_6_some thing.xml"), |
||||
Path("B01_1_some thing.tif"), |
||||
Path("C01_1_some thing.tif"), |
||||
Path("D01_1_some thing.tif"), |
||||
Path("E01_1_some thing.tif"), |
||||
Path("F01_1_some thing.tif"), |
||||
Path("G01_1_some thing.tif"), |
||||
Path("H01_1_some thing.tif"), |
||||
Path("I01_1_some thing.tif"), |
||||
Path("FF01_1_some thing.tif"), |
||||
Path("F21_1_some thing.tif"), |
||||
Path("F00_1_some thing.tif"), |
||||
Path("F0_1_some thing.tif"), |
||||
Path("F13_1_some thing.tif"), |
||||
Path("F08_some thing.tif"), |
||||
Path(".F09_1_some thing.tif"), |
||||
] |
||||
|
||||
|
||||
@pytest.mark.parametrize("sources", [sensovation_source_list, scienion_source_list]) |
||||
def test_parse_source_list_sensovation(sources): |
||||
from s2rename.helpers import RE_SENSOVATION, RE_SCIENION, parse_source_list |
||||
|
||||
regex = RE_SENSOVATION if sources == sensovation_source_list else RE_SCIENION |
||||
result = list(parse_source_list(sources, regex)) |
||||
|
||||
assert len(result) == 13 |
||||
assert {r.row for r in result} == set("ABCDEFGH") |
||||
assert {r.col for r in result} == {1, 2, 3, 10, 11, 12} |
||||
assert {r.channel for r in result} == set("123456") |
||||
assert {r.ext for r in result} == {".tif", ".csv", ".xml", ""} |
||||
assert {r.stem for r in result} == {"some thing", ""} |
||||
|
||||
|
||||
def test_get_source_list(): |
||||
from s2rename.helpers import get_source_list |
||||
|
||||
result = list(get_source_list(".")) |
||||
|
||||
assert {p.name for p in result} == { |
||||
"LICENSE", |
||||
"Pipfile", |
||||
"requirements_dev.txt", |
||||
"setup.cfg", |
||||
"Makefile", |
||||
"README.md", |
||||
"setup.py", |
||||
} |
||||
|
||||
|
||||
@pytest.mark.parametrize("path", ["/i/hope/this/doesnt/exist", "setup.py"]) |
||||
def test_get_source_list_raises_error(path): |
||||
from s2rename.helpers import get_source_list, S2IOError |
||||
|
||||
with pytest.raises(S2IOError): |
||||
get_source_list(path) |
||||
|
||||
|
||||
def test_get_source_list_with_type(): |
||||
from s2rename.helpers import get_source_list |
||||
|
||||
result = get_source_list(".", ".txt") |
||||
|
||||
assert {p.name for p in result} == {"requirements_dev.txt"} |
||||
|
||||
|
||||
def test_filter_source_list_type(): |
||||
from s2rename.helpers import filter_source_list |
||||
|
||||
result = list(filter_source_list(sensovation_source_list, ".csv")) |
||||
|
||||
assert len(result) == 1 |
||||
assert result[0].name == "some thing_A11_5.csv" |
||||
|
||||
|
||||
def test_get_new_name_simple(): |
||||
from s2rename.helpers import get_new_name, Renamable, template_sensovation |
||||
|
||||
r = Renamable( |
||||
path=Path("x.csv"), stem="some_name", row="A", col=2, channel=3, ext=".csv" |
||||
) |
||||
result = get_new_name(r, template_sensovation, digits=2) |
||||
|
||||
assert result == "some_name_A02_3.csv" |
||||
|
||||
|
||||
@pytest.mark.parametrize( |
||||
"tpl,stem,row,col,channel,digits,ext,expected", |
||||
[ |
||||
("sen", "some_name", "A", 1, 2, 1, ".xls", "some_name_A1_2.xls"), |
||||
("sen", "some_name", "A", 1, 2, 2, ".xls", "some_name_A01_2.xls"), |
||||
("sen", "some_name", "A", 1, 2, 3, ".xls", "some_name_A001_2.xls"), |
||||
("sen", "some_name", "B", 1, 2, 2, ".xls", "some_name_B01_2.xls"), |
||||
("sen", "some_name", "A", 2, 2, 2, ".xls", "some_name_A02_2.xls"), |
||||
("sen", "some_name", "A", 1, 3, 2, ".xls", "some_name_A01_3.xls"), |
||||
("sen", "some_name", "A", 1, 3, 2, ".tif", "some_name_A01_3.tif"), |
||||
("sen", "", "A", 1, 3, 2, ".xls", "A01_3.xls"), |
||||
("sci", "some_name", "A", 1, 2, 1, ".xls", "A1_2_some_name.xls"), |
||||
("sci", "some_name", "A", 1, 2, 2, ".xls", "A01_2_some_name.xls"), |
||||
("sci", "some_name", "A", 1, 2, 3, ".xls", "A001_2_some_name.xls"), |
||||
("sci", "some_name", "B", 1, 2, 2, ".xls", "B01_2_some_name.xls"), |
||||
("sci", "some_name", "A", 2, 2, 2, ".xls", "A02_2_some_name.xls"), |
||||
("sci", "some_name", "A", 1, 3, 2, ".xls", "A01_3_some_name.xls"), |
||||
("sci", "some_name", "A", 1, 3, 2, ".tif", "A01_3_some_name.tif"), |
||||
("sci", "", "A", 1, 3, 2, ".xls", "A01_3.xls"), |
||||
], |
||||
) |
||||
def test_get_new_name(tpl, stem, row, col, channel, digits, ext, expected): |
||||
from s2rename.helpers import ( |
||||
get_new_name, |
||||
Renamable, |
||||
template_sensovation, |
||||
template_scienion, |
||||
) |
||||
|
||||
path = Path("x" + ext) |
||||
r = Renamable(path=path, stem=stem, row=row, col=col, channel=channel, ext=ext) |
||||
tpl = template_sensovation if tpl == "sen" else template_scienion |
||||
result = get_new_name(r, tpl, digits=digits) |
||||
|
||||
assert result == expected |
||||
|
||||
|
||||
def test_rename_files(): |
||||
from s2rename.helpers import rename_files, Renamable, template_sensovation |
||||
|
||||
old_path = Path("old.txt") |
||||
new_path = Path("new_A001_2.xxx") |
||||
renamable_item = Renamable( |
||||
path=old_path, stem="new", row="A", col=1, channel=2, ext=".xxx" |
||||
) |
||||
with open(old_path, "w") as fh: |
||||
fh.write("\n") |
||||
assert old_path.is_file() |
||||
assert not new_path.is_file() |
||||
|
||||
rename_files([renamable_item], template_sensovation, 3) |
||||
|
||||
assert not old_path.is_file() |
||||
assert new_path.is_file() |
||||
|
||||
new_path.unlink() |
@ -1,111 +0,0 @@
@@ -1,111 +0,0 @@
|
||||
#!/usr/bin/env python |
||||
# -*- coding: utf-8 -*- |
||||
|
||||
"""Tests for `s2rename` package.""" |
||||
|
||||
import pytest |
||||
|
||||
from pathlib import Path |
||||
|
||||
|
||||
sensovation_source_list = [ |
||||
Path("some prefix thing_A1_1.tif"), |
||||
Path("some prefix thing_A02_2.tif"), |
||||
Path("A03_3.tif"), |
||||
Path("some prefix thing_A10_4"), |
||||
Path("some prefix thing_A11_5.csv"), |
||||
Path("some prefix thing_A12_6.xml"), |
||||
Path("some prefix thing_B01_1.tif"), |
||||
Path("some prefix thing_C01_1.tif"), |
||||
Path("some prefix thing_D01_1.tif"), |
||||
Path("some prefix thing_E01_1.tif"), |
||||
Path("some prefix thing_F01_1.tif"), |
||||
Path("some prefix thing_G01_1.tif"), |
||||
Path("some prefix thing_H01_1.tif"), |
||||
Path("some prefix thing_I01_1.tif"), |
||||
Path("some prefix thing_FF01_1.tif"), |
||||
Path("some prefix thing_F21_1.tif"), |
||||
Path("some prefix thing_F00_1.tif"), |
||||
Path("some prefix thing_F0_1.tif"), |
||||
Path("some prefix thing_F13_1.tif"), |
||||
Path("some prefix thing_F08.tif"), |
||||
Path(".some prefix thing_F09_1.tif"), |
||||
] |
||||
|
||||
scienion_source_list = [ |
||||
Path("A1_1_some suffix thing.tif"), |
||||
Path("A02_2_some suffix thing.tif"), |
||||
Path("A03_3.tif"), |
||||
Path("A10_3_some suffix thing"), |
||||
Path("A11_4_some suffix thing.csv"), |
||||
Path("A12_5_some suffix thing.xml"), |
||||
Path("B01_1_some suffix thing.tif"), |
||||
Path("C01_1_some suffix thing.tif"), |
||||
Path("D01_1_some suffix thing.tif"), |
||||
Path("E01_1_some suffix thing.tif"), |
||||
Path("F01_1_some suffix thing.tif"), |
||||
Path("G01_1_some suffix thing.tif"), |
||||
Path("H01_1_some suffix thing.tif"), |
||||
Path("I01_1_some suffix thing.tif"), |
||||
Path("FF01_1_some suffix thing.tif"), |
||||
Path("F21_1_some suffix thing.tif"), |
||||
Path("F00_1_some suffix thing.tif"), |
||||
Path("F0_1_some suffix thing.tif"), |
||||
Path("F13_1_some suffix thing.tif"), |
||||
Path("F08_some suffix thing.tif"), |
||||
Path(".F09_1_some suffix thing.tif"), |
||||
] |
||||
|
||||
|
||||
@pytest.mark.parametrize("sources", [sensovation_source_list, scienion_source_list]) |
||||
def test_parse_source_list_sensovation(sources): |
||||
from s2rename import s2rename |
||||
|
||||
if sources == sensovation_source_list: |
||||
regex = s2rename.RE_SENSOVATION |
||||
else: |
||||
regex = s2rename.RE_SCIENION |
||||
|
||||
result = list(s2rename.parse_source_list(sensovation_source_list, regex)) |
||||
assert len(result) == 14 |
||||
assert {r.row for r in result} == set("ABCDEFGH") |
||||
assert {r.col for r in result} == set([1, 2, 3, 9, 10, 11, 12]) |
||||
|
||||
|
||||
def test_get_source_list(): |
||||
from s2rename import s2rename |
||||
|
||||
result = list(s2rename.get_source_list(".")) |
||||
assert {p.name for p in result} == { |
||||
"LICENSE", |
||||
"Pipfile", |
||||
"requirements_dev.txt", |
||||
"setup.cfg", |
||||
"Makefile", |
||||
"README.md", |
||||
"setup.py", |
||||
} |
||||
|
||||
|
||||
@pytest.mark.parametrize("path", ["/i/hope/this/doesnt/exist", "setup.py"]) |
||||
def test_get_source_list_raises_error(path): |
||||
from s2rename import s2rename |
||||
|
||||
with pytest.raises(s2rename.S2IOError): |
||||
result = s2rename.get_source_list(path) |
||||
|
||||
|
||||
def test_get_source_list_with_type(): |
||||
from s2rename import s2rename |
||||
|
||||
result = s2rename.get_source_list(".", ".txt") |
||||
assert {p.name for p in result} == {"requirements_dev.txt"} |
||||
|
||||
|
||||
def test_filter_source_list_type(): |
||||
from s2rename import s2rename |
||||
|
||||
result = list(s2rename.filter_source_list(sensovation_source_list, ".csv")) |
||||
assert len(result) == 1 |
||||
assert result[0].name == "some prefix thing_A11_5.csv" |
||||
|
Reference in new issue