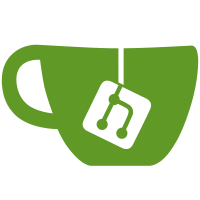
2 changed files with 2 additions and 54 deletions
@ -1,52 +0,0 @@ |
|||||||
import pathlib |
|
||||||
|
|
||||||
from tqdm import tqdm |
|
||||||
|
|
||||||
from .prescan import LABEL_SELECTED, LABEL_DISCARDED |
|
||||||
|
|
||||||
|
|
||||||
def stem_file_list(selected_files): |
|
||||||
return {pathlib.Path(filename).stem for filename in selected_files} |
|
||||||
|
|
||||||
|
|
||||||
def rename_color_coded_images(file_stems, settings): |
|
||||||
rename_pairs = [] |
|
||||||
for path in settings.colored_dir.iterdir(): |
|
||||||
if not path.stem.startswith("."): |
|
||||||
label = ( |
|
||||||
LABEL_SELECTED if path.stem in file_stems else LABEL_DISCARDED |
|
||||||
) |
|
||||||
new_name = path.with_name(f"{path.stem}_{label}{path.suffix}") |
|
||||||
rename_pairs.append((path, new_name)) |
|
||||||
return rename_pairs |
|
||||||
|
|
||||||
|
|
||||||
def sort_cut_images(file_stems, settings): |
|
||||||
sort_pairs = [] |
|
||||||
for path in settings.cuts_dir.iterdir(): |
|
||||||
if not path.stem.startswith("."): |
|
||||||
stem = path.stem[:-4] # removes the "_cut" suffix |
|
||||||
label = LABEL_SELECTED if stem in file_stems else LABEL_DISCARDED |
|
||||||
new_path = ( |
|
||||||
settings[f"cuts_{label}_dir"] |
|
||||||
/ f"{path.stem}_{label}{path.suffix}" |
|
||||||
) |
|
||||||
sort_pairs.append((path, new_path)) |
|
||||||
return sort_pairs |
|
||||||
|
|
||||||
|
|
||||||
def remove_cuts_dir(settings): |
|
||||||
for item in settings["cuts_dir"].iterdir(): |
|
||||||
item.unlink() |
|
||||||
settings["cuts_dir"].rmdir() |
|
||||||
|
|
||||||
|
|
||||||
def filesort_workflow(selected_files, settings): |
|
||||||
print("4/4: Sorting images") |
|
||||||
file_stems = stem_file_list(selected_files) |
|
||||||
cc_rename_pairs = rename_color_coded_images(file_stems, settings) |
|
||||||
cut_sort_pairs = sort_cut_images(file_stems, settings) |
|
||||||
file_pairs = cc_rename_pairs + cut_sort_pairs |
|
||||||
for old_path, new_path in tqdm(file_pairs): |
|
||||||
old_path.rename(new_path) |
|
||||||
remove_cuts_dir(settings) |
|
Loading…
Reference in new issue